Sometimes you need to shorten an existing cable by removing a section and reconnecting the two ends. Other times you might need to lengthen a cable by adding one or more sections. If you're doing this to a multi conductor cable, it's best to use the "Western Union" splice my grandfather taught me. This type of splice won't short-out (the condition in which conductors touch) if the insulation on your joint fails.
You will want to strip two to three times as much of the outer insulation as you normally would. On one end cut the red wire short, and the white wire long. On the other end cut the red wire long and the white wire short. Cut some heat-shrink tubing to go over the inner conductors. Also cut a larger piece to go over the whole thing, slide it over one or the other of the pieces of cable, and slide it well out of the way. Don't forget to do this, because after you've made the connection, you won't get be able to do get the heat-shrink onto the cable. Now cut some smaller heat-shrink tubing for the inner conductors and slide them over each of the longer wires. Make sure these are far away from the joint so the heat from soldering won't shrink them and prevent them from fitting over the joint.
Twist the wires together facing each other such that the joint isn't much thicker than the original wire. If you twist them facing the same direction (like a zipper), you might not be able to get the heat shrink over them, and if you do, the tubing will look like a python that's swallowed a pig. If the conductor is going to poke through the heat-shrink and cause a short, it's going to be where it's stretched thin over the big solder blob.
Like this:
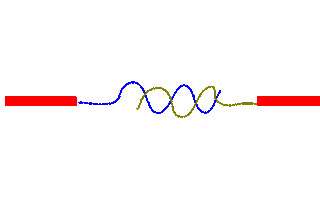
Not like this:
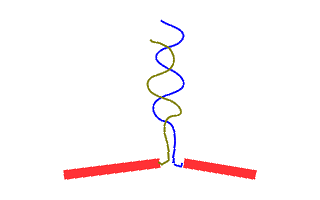
Apply heat, then solder to the joints. After the joints have cooled, slide the heat shrink over them and apply heat. After that's cooled down, slide the large heat-shrink over the whole thing and apply heat to complete the job.